
In today's rapidly advancing technological landscape, natural language processing and comprehension have become essential components of everyday life. Leading the charge in this arena is OpenAI's ChatGPT API, renowned for its exceptional ability to understand and interact with human language. Imagine elevating ChatGPT's functionality to new heights, enabling it to carry out specific tasks based on commands given in natural language. This article aims to shed light on the potential of incorporating function calling into the ChatGPT API, thereby enhancing its utility. I will illustrate through practical examples how such extensions can unlock a myriad of opportunities and applications.
Function Calling in ChatGPT API
Function Calling and Its Potential
Function calling within the ChatGPT API involves the ability to invoke custom functions based on natural language input. This transformative feature allows you to turn plain text queries into actionable commands, opening up a world of possibilities across diverse domains. Whether you're in the realms of software development, data analysis, or even running a startup, the ability to have a natural language conversation with your applications can streamline processes and enhance user experiences.
Solutions for Natural Language Converted to Instructions
- Automating Repetitive Tasks: You can automate repetitive and time-consuming tasks by creating functions that understand and execute specific instructions. For instance, you could use ChatGPT to manage your email, schedule meetings, or update your to-do list without ever having to leave your messaging platform.
- Data Analysis and Reporting: ChatGPT can be a valuable tool for data analysts. By integrating it with data visualization tools or databases, you can ask complex questions about your data and receive clear, comprehensible reports.
- Customer Support Chatbots: Enhance your customer support by enabling ChatGPT to understand user queries and provide relevant solutions. From troubleshooting technical issues to answering frequently asked questions, your chatbot can be more effective and user-friendly.
Real-World Examples of Integrations
Let's explore practical cases to demonstrate the effectiveness of augmenting ChatGPT's functions. To proceed with the examples below, ensure you have NodeJS and npm/yarn/pnpm installed.
The OpenAI NodeJS library offers a user-friendly helper for function invocation. Essentially, it requires you to specify a list of your functions along with their schema, and based on the chat input provided, the appropriate function will be executed.
Example 1: Replicate - Running AI Models in the Cloud
Replicate is a SaaS platform that allows users to run AI models in the cloud, for example, enabling audio, image, and video generation, and more. By integrating ChatGPT with Replicate, you can create a seamless interface for generating multimedia content. For instance, you can instruct ChatGPT with a simple query like "Generate a photorealistic image of a cat walking on the moon in the red hat" and it will run Stable Diffusion model via Replicate's API.
import readline from 'readline';
import OpenAI from 'openai';
import Replicate from 'replicate';
// Inteface for reading user input from the console
const rl = readline.createInterface({
input: process.stdin,
output: process.stdout,
});
const openai = new OpenAI({
apiKey: process.env.OPENAI_API_KEY
});
const replicate = new Replicate({
auth: process.env.REPLICATE_API_TOKEN
});
async function main() {
const content = await new Promise(resolve => {
rl.question('Your request: ', resolve);
});
const runner = openai.beta.chat.completions
.runTools({
model: 'gpt-3.5-turbo',
messages: [
{
role: 'user',
content
}
],
tools: [
{
type: 'function',
function: {
function: generateImage,
parse: JSON.parse,
parameters: {
type: 'object',
properties: {
prompt: {
type: 'string'
},
},
},
}
}
]
});
const result = await runner.finalContent();
rl.write(result);
rl.close();
};
async function generateImage({ prompt }) {
console.log('Generating image...');
const output = await replicate.run(
// Latest version can be found at https://replicate.com/stability-ai/sdxl/api
"stability-ai/sdxl:39ed52f2a78e934b3ba6e2a89f5b1c712de7dfea535525255b1aa35c5565e08b",
{
input: {
prompt
}
}
);
return output[0];
}
main();
Here is an execution demo:
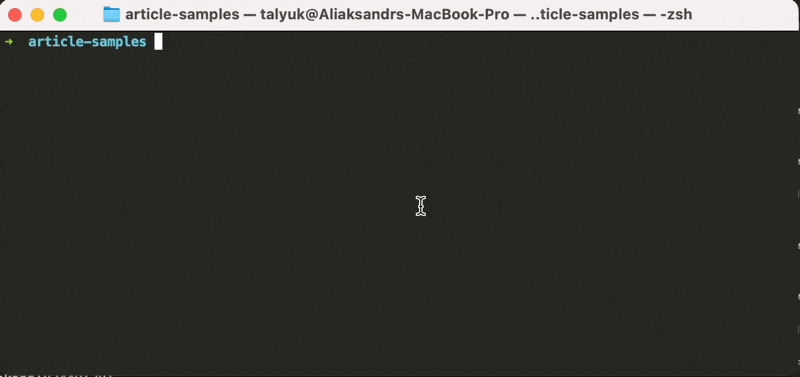
Since URLs that Replicate returns are temporary, I'm attaching the result here:
Generated image

This way, one of the options of instrumenting ChatGPT with Replicate is a chatbot for content creators, providing access to a large variety of models - video generation, audio generation, 3D models generation, and lots more.
Example 2: Foursquare Integration - Finding the Perfect Restaurant
By connecting ChatGPT with the Foursquare API, you can transform queries like "Where can I eat octopus tonight in SF?" into actionable commands that search for restaurants meeting these criteria. This integration provides users with tailored recommendations for their dining preferences.
Consider a traveler in an unfamiliar city. They ask ChatGPT for dining suggestions, and it uses the Foursquare API to identify nearby restaurants with octopus dishes. Users can even specify other preferences like cuisine type and price range, making it a personalized dining assistant.
import readline from 'readline';
import OpenAI from 'openai';
// Inteface for reading user input from the console
const rl = readline.createInterface({
input: process.stdin,
output: process.stdout,
});
const openai = new OpenAI({
apiKey: process.env.OPENAI_API_KEY
});
async function main() {
const content = await new Promise(resolve => {
rl.question('Your request: ', resolve);
});
const runner = openai.beta.chat.completions
.runTools({
model: 'gpt-3.5-turbo',
messages: [
{
role: 'user',
content
}
],
tools: [
{
type: 'function',
function: {
function: searchPlace,
parse: JSON.parse,
parameters: {
type: 'object',
// All available properties might be found at https://location.foursquare.com/developer/reference/place-search
properties: {
query: {
type: 'string',
description: 'A string to be matched against all content for this place, including but not limited to venue name, category, telephone number, taste, and tips.'
},
open_at: {
type: 'string',
description: 'Support local day and local time requests through this parameter. To be specified as DOWTHHMM (e.g., 1T2130), where DOW is the day number 1-7 (Monday = 1, Sunday = 7) and time is in 24 hour format.'
},
near: {
type: 'string',
description: 'A string naming a locality in the world (e.g., "Chicago, IL")'
}
},
},
}
}
]
})
const result = await runner.finalContent();
rl.write(result);
rl.close();
};
async function searchPlace(params) {
console.log('Searching place...', params);
const response = await fetch(
'https://api.foursquare.com/v3/places/search?' + new URLSearchParams(params),
{
method: 'GET',
headers: {
'Accept': 'application/json',
'Authorization': process.env.FOURSQUARE_API_TOKEN
},
}
);
const output = await response.json();
return output;
}
main();
Here is an execution demo:
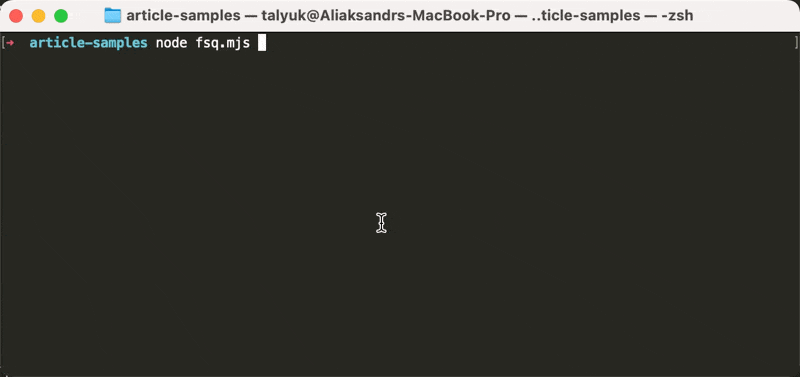
Instrumenting ChatGPT with Foursquare API allows to create a personalized chatbot for searching restaurants using natural language. If you're building a personal assistant, it might be the next feature!
Example 3: DevOps Automation with DigitalOcean
DevOps professionals can streamline infrastructure management by using ChatGPT to generate commands for DigitalOcean's API. A prompt like "Setup auto-scaling DB cluster in SFO3 region" can be converted into API calls that configure the desired infrastructure, simplifying the DevOps workflow.
In this scenario, a DevOps engineer issues high-level infrastructure commands in plain language. ChatGPT translates these commands into API calls that provision and configure servers, databases, and other resources. This seamless interaction boosts efficiency and minimizes the scope for manual errors.
import readline from 'readline';
import OpenAI from 'openai';
// Inteface for reading user input from the console
const rl = readline.createInterface({
input: process.stdin,
output: process.stdout,
});
const openai = new OpenAI({
apiKey: process.env.OPENAI_API_KEY
});
async function main() {
const content = await new Promise(resolve => {
rl.question('Your request: ', resolve);
});
const runner = openai.beta.chat.completions
.runTools({
model: 'gpt-3.5-turbo',
messages: [
{
role: 'user',
content
}
],
tools: [
{
type: 'function',
function: {
function: createDatabaseCluster,
parse: JSON.parse,
parameters: {
type: 'object',
// All properties might be found at https://docs.digitalocean.com/reference/api/api-reference/#operation/databases_create_cluster
properties: {
name: {
type: 'string',
description: 'A unique, human-readable name referring to a database cluster.'
},
engine: {
type: 'string',
description: 'A slug representing the database engine used for the cluster. The possible values are: "pg" for PostgreSQL, "mysql" for MySQL, "redis" for Redis, "mongodb" for MongoDB, and "kafka" for Kafka.'
},
num_nodes: {
type: 'number',
default: 1,
description: 'The number of nodes in the database cluster.'
},
size: {
type: 'string',
description: 'The slug identifier representing the size of the nodes in the database cluster.'
},
region: {
type: 'string',
description: 'The slug identifier for the region where the database cluster is located.'
},
},
},
}
}
]
})
const result = await runner.finalContent();
rl.write(result);
rl.close();
};
async function createDatabaseCluster(params) {
console.log('Creating database cluster...', params);
const response = await fetch(
'https://api.digitalocean.com/v2/databases',
{
method: 'POST',
headers: {
'Accept': 'application/json',
'Authorization': `Bearer ${process.env.DIGITALOCEAN_API_TOKEN}`
},
body: JSON.stringify(params)
}
);
const output = await response.json();
return output;
}
main();
Execution demo that showcases cluster being created:
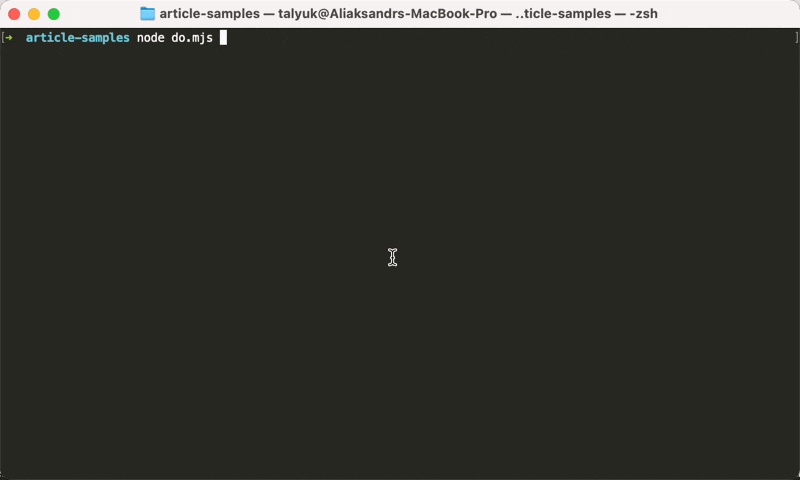
If I navigate to the Digitalocean Console, I see the newly created cluster with the requested configuration:

The Power of Function Calling
Function calling in ChatGPT API is a game-changer that can simplify and extend your productivity. It empowers you to communicate with your applications in natural language, reducing the need for complex interfaces and manual interactions. By leveraging this technology, you can uncover new product ideas, enhance user experiences, and even run your startup more efficiently. The possibilities are limitless, and the only constraint is your imagination. Welcome to the future of natural language for custom instructions - it's here, and it's transformative. Function calling in ChatGPT API is not just a tool; it's a catalyst for innovation, opening doors to a world where communication with machines becomes as natural as a conversation with a friend.